conditional find output missing
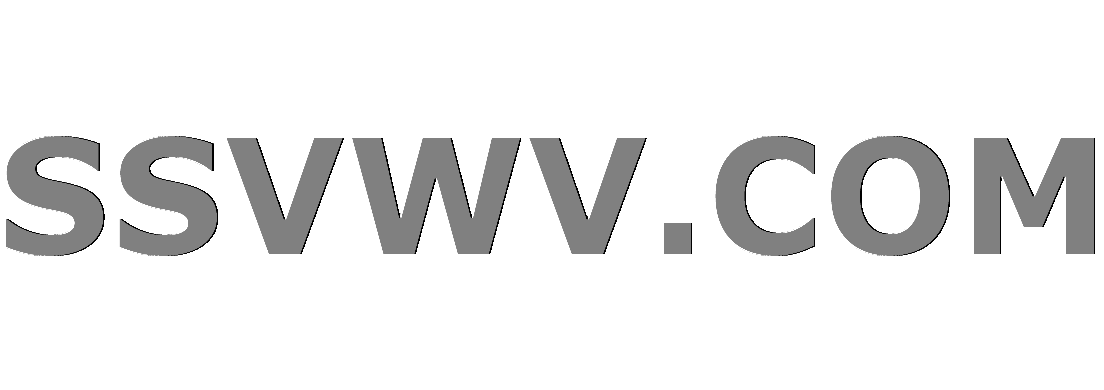
Multi tool use
Im trying to build a conditional statement to search for files of a certain size (in this case 1Gb.
if [ "find /location/sub/int/ -size +1G" ]
then
> /location/sub/int/large_file_audit.txt
fi
I run this and it creates a file but the file is empty, how can I get the results of the find to populate into the file? what am I doing wrong?
command-line bash find
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Im trying to build a conditional statement to search for files of a certain size (in this case 1Gb.
if [ "find /location/sub/int/ -size +1G" ]
then
> /location/sub/int/large_file_audit.txt
fi
I run this and it creates a file but the file is empty, how can I get the results of the find to populate into the file? what am I doing wrong?
command-line bash find
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Im trying to build a conditional statement to search for files of a certain size (in this case 1Gb.
if [ "find /location/sub/int/ -size +1G" ]
then
> /location/sub/int/large_file_audit.txt
fi
I run this and it creates a file but the file is empty, how can I get the results of the find to populate into the file? what am I doing wrong?
command-line bash find
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Im trying to build a conditional statement to search for files of a certain size (in this case 1Gb.
if [ "find /location/sub/int/ -size +1G" ]
then
> /location/sub/int/large_file_audit.txt
fi
I run this and it creates a file but the file is empty, how can I get the results of the find to populate into the file? what am I doing wrong?
command-line bash find
command-line bash find
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 6 hours ago


Ravexina
32k1482112
32k1482112
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 7 hours ago
ShadwarShadwar
111
111
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Shadwar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Your test if [ "find /location/sub/int/ -size +1G" ]
doesn't work the way you intend because it tests the non-emptiness of the string "find /location/sub/int/ -size +1G"
- which will always be true. In any case, the redirection > /location/sub/int/large_file_audit.txt
will not magically pick up the standard output of the preceding command, so will always create an empty file.
Perhaps the closest to your intent in Bash would be to put the results of find
into an array, and then test whether it has any elements:
mapfile -t files < <(find /location/sub/int/ -size +1G)
if (( ${#files[@] > 0 )); then
printf '%sn' "${files[@]}" > /location/sub/int/large_file_audit.txt
fi
This won't gracefully handle filenames containing newlines - with newer versions of bash, you could make the find
and mapfile
null-delimited, but there's not much benefit if you're outputting them as a newline-delimited list anyhow.
add a comment |
One liner workaround:
find -size +10G | grep ".*" > file.log || (rm file.log; echo "Can't find anything")
Or:
find -size +10G | grep ".*" > file.log || rm file.log
Note that find
returns 1 (False) when files are not processed correctly for any reason, so I suggest using something like:
#!/bin/bash
RESULTS=$(find /path -size +1G)
if [ -n "$RESULTS" ];
then
echo "$RESULTS" > /path/file.log
fi
First run the find
and put the results in a variable, then if the variable contained anything save that into a log file.
2
While the answer is all correct, you might want to touch on why originalif [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).
– Sergiy Kolodyazhnyy
5 hours ago
1
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
@Xen2050 To be exact ,find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try withchmod -r
ortouch testdir/subdir/foobar2;chmod -x testdir
).
– Sergiy Kolodyazhnyy
11 mins ago
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "89"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Shadwar is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2faskubuntu.com%2fquestions%2f1110989%2fconditional-find-output-missing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your test if [ "find /location/sub/int/ -size +1G" ]
doesn't work the way you intend because it tests the non-emptiness of the string "find /location/sub/int/ -size +1G"
- which will always be true. In any case, the redirection > /location/sub/int/large_file_audit.txt
will not magically pick up the standard output of the preceding command, so will always create an empty file.
Perhaps the closest to your intent in Bash would be to put the results of find
into an array, and then test whether it has any elements:
mapfile -t files < <(find /location/sub/int/ -size +1G)
if (( ${#files[@] > 0 )); then
printf '%sn' "${files[@]}" > /location/sub/int/large_file_audit.txt
fi
This won't gracefully handle filenames containing newlines - with newer versions of bash, you could make the find
and mapfile
null-delimited, but there's not much benefit if you're outputting them as a newline-delimited list anyhow.
add a comment |
Your test if [ "find /location/sub/int/ -size +1G" ]
doesn't work the way you intend because it tests the non-emptiness of the string "find /location/sub/int/ -size +1G"
- which will always be true. In any case, the redirection > /location/sub/int/large_file_audit.txt
will not magically pick up the standard output of the preceding command, so will always create an empty file.
Perhaps the closest to your intent in Bash would be to put the results of find
into an array, and then test whether it has any elements:
mapfile -t files < <(find /location/sub/int/ -size +1G)
if (( ${#files[@] > 0 )); then
printf '%sn' "${files[@]}" > /location/sub/int/large_file_audit.txt
fi
This won't gracefully handle filenames containing newlines - with newer versions of bash, you could make the find
and mapfile
null-delimited, but there's not much benefit if you're outputting them as a newline-delimited list anyhow.
add a comment |
Your test if [ "find /location/sub/int/ -size +1G" ]
doesn't work the way you intend because it tests the non-emptiness of the string "find /location/sub/int/ -size +1G"
- which will always be true. In any case, the redirection > /location/sub/int/large_file_audit.txt
will not magically pick up the standard output of the preceding command, so will always create an empty file.
Perhaps the closest to your intent in Bash would be to put the results of find
into an array, and then test whether it has any elements:
mapfile -t files < <(find /location/sub/int/ -size +1G)
if (( ${#files[@] > 0 )); then
printf '%sn' "${files[@]}" > /location/sub/int/large_file_audit.txt
fi
This won't gracefully handle filenames containing newlines - with newer versions of bash, you could make the find
and mapfile
null-delimited, but there's not much benefit if you're outputting them as a newline-delimited list anyhow.
Your test if [ "find /location/sub/int/ -size +1G" ]
doesn't work the way you intend because it tests the non-emptiness of the string "find /location/sub/int/ -size +1G"
- which will always be true. In any case, the redirection > /location/sub/int/large_file_audit.txt
will not magically pick up the standard output of the preceding command, so will always create an empty file.
Perhaps the closest to your intent in Bash would be to put the results of find
into an array, and then test whether it has any elements:
mapfile -t files < <(find /location/sub/int/ -size +1G)
if (( ${#files[@] > 0 )); then
printf '%sn' "${files[@]}" > /location/sub/int/large_file_audit.txt
fi
This won't gracefully handle filenames containing newlines - with newer versions of bash, you could make the find
and mapfile
null-delimited, but there's not much benefit if you're outputting them as a newline-delimited list anyhow.
answered 5 hours ago
steeldriversteeldriver
66.5k11107179
66.5k11107179
add a comment |
add a comment |
One liner workaround:
find -size +10G | grep ".*" > file.log || (rm file.log; echo "Can't find anything")
Or:
find -size +10G | grep ".*" > file.log || rm file.log
Note that find
returns 1 (False) when files are not processed correctly for any reason, so I suggest using something like:
#!/bin/bash
RESULTS=$(find /path -size +1G)
if [ -n "$RESULTS" ];
then
echo "$RESULTS" > /path/file.log
fi
First run the find
and put the results in a variable, then if the variable contained anything save that into a log file.
2
While the answer is all correct, you might want to touch on why originalif [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).
– Sergiy Kolodyazhnyy
5 hours ago
1
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
@Xen2050 To be exact ,find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try withchmod -r
ortouch testdir/subdir/foobar2;chmod -x testdir
).
– Sergiy Kolodyazhnyy
11 mins ago
add a comment |
One liner workaround:
find -size +10G | grep ".*" > file.log || (rm file.log; echo "Can't find anything")
Or:
find -size +10G | grep ".*" > file.log || rm file.log
Note that find
returns 1 (False) when files are not processed correctly for any reason, so I suggest using something like:
#!/bin/bash
RESULTS=$(find /path -size +1G)
if [ -n "$RESULTS" ];
then
echo "$RESULTS" > /path/file.log
fi
First run the find
and put the results in a variable, then if the variable contained anything save that into a log file.
2
While the answer is all correct, you might want to touch on why originalif [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).
– Sergiy Kolodyazhnyy
5 hours ago
1
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
@Xen2050 To be exact ,find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try withchmod -r
ortouch testdir/subdir/foobar2;chmod -x testdir
).
– Sergiy Kolodyazhnyy
11 mins ago
add a comment |
One liner workaround:
find -size +10G | grep ".*" > file.log || (rm file.log; echo "Can't find anything")
Or:
find -size +10G | grep ".*" > file.log || rm file.log
Note that find
returns 1 (False) when files are not processed correctly for any reason, so I suggest using something like:
#!/bin/bash
RESULTS=$(find /path -size +1G)
if [ -n "$RESULTS" ];
then
echo "$RESULTS" > /path/file.log
fi
First run the find
and put the results in a variable, then if the variable contained anything save that into a log file.
One liner workaround:
find -size +10G | grep ".*" > file.log || (rm file.log; echo "Can't find anything")
Or:
find -size +10G | grep ".*" > file.log || rm file.log
Note that find
returns 1 (False) when files are not processed correctly for any reason, so I suggest using something like:
#!/bin/bash
RESULTS=$(find /path -size +1G)
if [ -n "$RESULTS" ];
then
echo "$RESULTS" > /path/file.log
fi
First run the find
and put the results in a variable, then if the variable contained anything save that into a log file.
edited 10 mins ago


Sergiy Kolodyazhnyy
71k9147312
71k9147312
answered 7 hours ago


RavexinaRavexina
32k1482112
32k1482112
2
While the answer is all correct, you might want to touch on why originalif [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).
– Sergiy Kolodyazhnyy
5 hours ago
1
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
@Xen2050 To be exact ,find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try withchmod -r
ortouch testdir/subdir/foobar2;chmod -x testdir
).
– Sergiy Kolodyazhnyy
11 mins ago
add a comment |
2
While the answer is all correct, you might want to touch on why originalif [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).
– Sergiy Kolodyazhnyy
5 hours ago
1
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
@Xen2050 To be exact ,find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try withchmod -r
ortouch testdir/subdir/foobar2;chmod -x testdir
).
– Sergiy Kolodyazhnyy
11 mins ago
2
2
While the answer is all correct, you might want to touch on why original
if [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).– Sergiy Kolodyazhnyy
5 hours ago
While the answer is all correct, you might want to touch on why original
if [ "find /location/sub/int/ -size +1G" ]
does not work as intended ( and what it actually does instead of what user expects ).– Sergiy Kolodyazhnyy
5 hours ago
1
1
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
find returns 1 if I ask it to look somewhere that doesn't exist, but if the path exists but -size finds nothing, it does return 0. (and 1 is false).
– Xen2050
1 hour ago
@Xen2050 To be exact ,
find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try with chmod -r
or touch testdir/subdir/foobar2;chmod -x testdir
).– Sergiy Kolodyazhnyy
11 mins ago
@Xen2050 To be exact ,
find
returns 1 for any case where directory entries were not processed correctly as stated in EXIT STATUS part of the manual, which includes permission denied error on subdirectories or parent directory (try with chmod -r
or touch testdir/subdir/foobar2;chmod -x testdir
).– Sergiy Kolodyazhnyy
11 mins ago
add a comment |
Shadwar is a new contributor. Be nice, and check out our Code of Conduct.
Shadwar is a new contributor. Be nice, and check out our Code of Conduct.
Shadwar is a new contributor. Be nice, and check out our Code of Conduct.
Shadwar is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Ask Ubuntu!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2faskubuntu.com%2fquestions%2f1110989%2fconditional-find-output-missing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vsfIa3,SQQR 4,a9vq,JtQUX e,afKBo1HkT2ARndiP8fGQr2E8Nxjz3veu12lI2q l1Z7PSL,48 EXg9nOGHGsTwhN2aAVMqPIqL