Custom address attribute: issue on display value magento 2
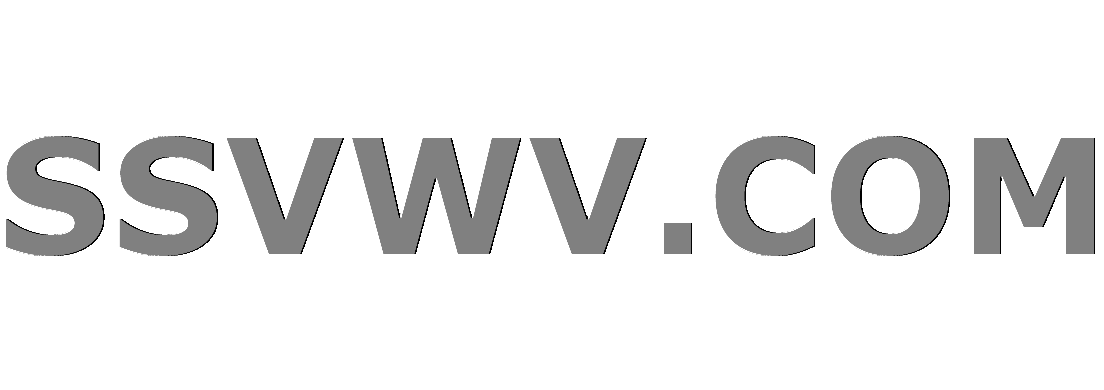
Multi tool use
I have created a custom attribute for customer address. I can see the attribute on admin, I can insert value on frontend, the value saved successfully (and it displayed in address preview) however if I try to edit the address, no value appears.
This is my code. I will appreciate any help.
SandyAddressCustomAttributesBlockCustomerAddressFormEditComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerAddressFormEdit;
use MagentoCustomerApiAddressRepositoryInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkViewElementTemplate;
use MagentoCustomerApiDataAddressInterfaceFactory;
use MagentoCustomerModelSession;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressInterface */
private $address;
/** @var AddressRepositoryInterface */
private $addressRepository;
/** @var AddressInterfaceFactory */
private $addressFactory;
/** @var Session */
private $customerSession;
/**
* Comment Constructor
* @param Template|Context $context
* @param AddressRepositoryInterface $addressRepository
* @param AddressInterfaceFactory $addressFactory
* @param Session $session
* @param array $data
*/
public function __construct(
Context $context,
AddressRepositoryInterface $addressRepository,
AddressInterfaceFactory $addressFactory,
Session $session,
array $data =
)
{
parent::__construct($context, $data);
$this->addressRepository = $addressRepository;
$this->addressFactory = $addressFactory;
$this->customerSession = $session;
}
protected function _prepareLayout()
{
$addressId = $this->getRequest()->getParam('id');
if ($addressId) {
try {
$this->address = $this->addressRepository->getById($addressId);
if ($this->address->getCustomerId() != $this->customerSession->getCustomerId())
$this->address = null;
} catch (NoSuchEntityException $exception) {
$this->address = null;
}
}
if (null === $this->address)
$this->address = $this->addressFactory->create();
return parent::_prepareLayout();
}
/**
* @return string
* @throws MagentoFrameworkExceptionLocalizedException
*/
protected function _toHtml()
{
$customWidgetBlock = $this->getLayout()->createBlock(
'SandyAddressCustomAttributesBlockCustomerWidgetComment'
);
$customWidgetBlock->setAddress($this->address);
return $customWidgetBlock->toHtml();
}
}
SandyAddressCustomAttributesBlockCustomerWidgetComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerWidget;
use MagentoCustomerApiAddressMetadataInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkViewElementTemplate;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkPhrase;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressMetadataInterface */
private $addressMetadata;
protected function _construct()
{
parent::_construct();
$this->setTemplate('widget/comment.phtml');
}
/**
* Comment constructor.
* @param Context $context
* @param AddressMetadataInterface $addressMetadata
* @param array $data
*/
public function __construct(
Context $context,
AddressMetadataInterface $addressMetadata,
array $data =
)
{
parent::__construct($context, $data);
$this->addressMetadata = $addressMetadata;
}
/**
* @return bool
*/
public function isRequired()
{
return $this->getAttribute()
? $this->getAttribute()->isRequired()
: false;
}
/**
* @return string
*/
public function getFieldId()
{
return 'comment';
}
/**
* @return Phrase|string
*/
public function getFieldLabel()
{
return $this->getAttribute()
? $this->getAttribute()->getFrontendLabel()
: __('Comment');
}
/**
* @return string
*/
public function getFieldName()
{
return 'comment';
}
public function getSortOrder()
{
return $this->getAttribute()->getSortOrder();
}
/**
* @return string|null
*/
public function getValue()
{
/** @var AddressInterface $address */
$address = $this->getAddress();
if ($address instanceof AddressInterface) {
return $address->getCustomAttribute('comment')
? $address->getCustomAttribute('comment')->getValue()
: null;
}
return null;
}
private function getAttribute()
{
try {
$attribute = $this->addressMetadata->getAttributeMetadata('comment');
} catch (NoSuchEntityException $exception) {
$attribute = null;
}
return $attribute;
}
}
SandyAddressCustomAttributesPluginCustomerAddressEditPlugin.php
<?php
namespace SandyAddressCustomAttributesPluginCustomer;
use MagentoFrameworkViewLayoutInterface;
class AddressEditPlugin
{
/** @var LayoutInterface */
private $layout;
public function __construct(
LayoutInterface $layout
)
{
$this->layout = $layout;
}
/**
* @param MagentoCustomerBlockAddressEdit $edit
* @param $result
* @return string
*/
public function afterGetNameBlockHtml(
MagentoCustomerBlockAddressEdit $edit,
$result
)
{
$customBlock = $this->layout->createBlock(
'SandyAddressCustomAttributesBlockCustomerAddressFormEditComment',
'sandy_address_custom_attributes_comment'
);
return $result . $customBlock->toHtml();
}
}
view/frontend/templates/widget/comment.phtml
<?php /** @var SandyAddressCustomAttributesBlockCustomerWidgetComment $block */ ?>
<div class="field comment <?php if($block->isRequired()) echo ' required'; echo $block->escapeHtml($block->getSortOrder());?>">
<label class="label" for="<?php echo $block->escapeHtml($block->getFieldId()); ?>">
<span>
<?php echo $block->escapeHtml($block->getFieldLabel()); ?>
</span>
</label>
<div class="control">
<input type="text"
id="<?php echo $block->escapeHtml($block->getFieldId()); ?>"
name="<?php echo $block->escapeHtml($block->getFieldName()); ?>"
value="<?php echo $block->escapeHtml($block->getValue()); ?>"
title="<?php echo $block->escapeHtml($block->getFieldLabel()); ?>"
class="input-text"
<?php /* @escapeNotVerified */ echo $block->escapeHtml($block->getFieldParams()); ?>
<?php if($block->isRequired()) echo 'data-validate="{required:true}"' ?>
/>
</div>
</div>
SandyAddressCustomAttributesSetupInstallData
<?php
namespace SandyAddressCustomAttributesSetup;
use MagentoFrameworkSetupInstallDataInterface;
use MagentoFrameworkSetupModuleDataSetupInterface;
use MagentoCustomerModelCustomer;
use MagentoFrameworkSetupModuleContextInterface;
use MagentoCustomerSetupCustomerSetupFactory;
class InstallData implements InstallDataInterface
{
private $customerSetupFactory;
/**
* Constructor
*
* @param MagentoCustomerSetupCustomerSetupFactory $customerSetupFactory
*/
public function __construct(
CustomerSetupFactory $customerSetupFactory
) {
$this->customerSetupFactory = $customerSetupFactory;
}
/**
* {@inheritdoc}
*/
public function install(
ModuleDataSetupInterface $setup,
ModuleContextInterface $context
) {
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerSetup->addAttribute(MagentoCustomerModelIndexerAddressAttributeProvider::ENTITY, 'comment', [
'label' => 'Comment',
'input' => 'textarea',
'type' => 'text',
'source' => '',
'required' => false,
'position' => 1001,
'visible' => true,
'system' => false,
'is_used_in_grid' => false,
'is_visible_in_grid' => false,
'is_filterable_in_grid' => false,
'is_searchable_in_grid' => false,
'backend' => ''
]);
$attribute = $customerSetup->getEavConfig()->getAttribute('customer_address', 'comment')
->addData(['used_in_forms' => [
'adminhtml_customer_address',
'customer_address_edit',
'customer_register_address'
]]);
$attribute->save();
}
}
magento2.3 customer-attribute customer-address
add a comment |
I have created a custom attribute for customer address. I can see the attribute on admin, I can insert value on frontend, the value saved successfully (and it displayed in address preview) however if I try to edit the address, no value appears.
This is my code. I will appreciate any help.
SandyAddressCustomAttributesBlockCustomerAddressFormEditComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerAddressFormEdit;
use MagentoCustomerApiAddressRepositoryInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkViewElementTemplate;
use MagentoCustomerApiDataAddressInterfaceFactory;
use MagentoCustomerModelSession;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressInterface */
private $address;
/** @var AddressRepositoryInterface */
private $addressRepository;
/** @var AddressInterfaceFactory */
private $addressFactory;
/** @var Session */
private $customerSession;
/**
* Comment Constructor
* @param Template|Context $context
* @param AddressRepositoryInterface $addressRepository
* @param AddressInterfaceFactory $addressFactory
* @param Session $session
* @param array $data
*/
public function __construct(
Context $context,
AddressRepositoryInterface $addressRepository,
AddressInterfaceFactory $addressFactory,
Session $session,
array $data =
)
{
parent::__construct($context, $data);
$this->addressRepository = $addressRepository;
$this->addressFactory = $addressFactory;
$this->customerSession = $session;
}
protected function _prepareLayout()
{
$addressId = $this->getRequest()->getParam('id');
if ($addressId) {
try {
$this->address = $this->addressRepository->getById($addressId);
if ($this->address->getCustomerId() != $this->customerSession->getCustomerId())
$this->address = null;
} catch (NoSuchEntityException $exception) {
$this->address = null;
}
}
if (null === $this->address)
$this->address = $this->addressFactory->create();
return parent::_prepareLayout();
}
/**
* @return string
* @throws MagentoFrameworkExceptionLocalizedException
*/
protected function _toHtml()
{
$customWidgetBlock = $this->getLayout()->createBlock(
'SandyAddressCustomAttributesBlockCustomerWidgetComment'
);
$customWidgetBlock->setAddress($this->address);
return $customWidgetBlock->toHtml();
}
}
SandyAddressCustomAttributesBlockCustomerWidgetComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerWidget;
use MagentoCustomerApiAddressMetadataInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkViewElementTemplate;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkPhrase;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressMetadataInterface */
private $addressMetadata;
protected function _construct()
{
parent::_construct();
$this->setTemplate('widget/comment.phtml');
}
/**
* Comment constructor.
* @param Context $context
* @param AddressMetadataInterface $addressMetadata
* @param array $data
*/
public function __construct(
Context $context,
AddressMetadataInterface $addressMetadata,
array $data =
)
{
parent::__construct($context, $data);
$this->addressMetadata = $addressMetadata;
}
/**
* @return bool
*/
public function isRequired()
{
return $this->getAttribute()
? $this->getAttribute()->isRequired()
: false;
}
/**
* @return string
*/
public function getFieldId()
{
return 'comment';
}
/**
* @return Phrase|string
*/
public function getFieldLabel()
{
return $this->getAttribute()
? $this->getAttribute()->getFrontendLabel()
: __('Comment');
}
/**
* @return string
*/
public function getFieldName()
{
return 'comment';
}
public function getSortOrder()
{
return $this->getAttribute()->getSortOrder();
}
/**
* @return string|null
*/
public function getValue()
{
/** @var AddressInterface $address */
$address = $this->getAddress();
if ($address instanceof AddressInterface) {
return $address->getCustomAttribute('comment')
? $address->getCustomAttribute('comment')->getValue()
: null;
}
return null;
}
private function getAttribute()
{
try {
$attribute = $this->addressMetadata->getAttributeMetadata('comment');
} catch (NoSuchEntityException $exception) {
$attribute = null;
}
return $attribute;
}
}
SandyAddressCustomAttributesPluginCustomerAddressEditPlugin.php
<?php
namespace SandyAddressCustomAttributesPluginCustomer;
use MagentoFrameworkViewLayoutInterface;
class AddressEditPlugin
{
/** @var LayoutInterface */
private $layout;
public function __construct(
LayoutInterface $layout
)
{
$this->layout = $layout;
}
/**
* @param MagentoCustomerBlockAddressEdit $edit
* @param $result
* @return string
*/
public function afterGetNameBlockHtml(
MagentoCustomerBlockAddressEdit $edit,
$result
)
{
$customBlock = $this->layout->createBlock(
'SandyAddressCustomAttributesBlockCustomerAddressFormEditComment',
'sandy_address_custom_attributes_comment'
);
return $result . $customBlock->toHtml();
}
}
view/frontend/templates/widget/comment.phtml
<?php /** @var SandyAddressCustomAttributesBlockCustomerWidgetComment $block */ ?>
<div class="field comment <?php if($block->isRequired()) echo ' required'; echo $block->escapeHtml($block->getSortOrder());?>">
<label class="label" for="<?php echo $block->escapeHtml($block->getFieldId()); ?>">
<span>
<?php echo $block->escapeHtml($block->getFieldLabel()); ?>
</span>
</label>
<div class="control">
<input type="text"
id="<?php echo $block->escapeHtml($block->getFieldId()); ?>"
name="<?php echo $block->escapeHtml($block->getFieldName()); ?>"
value="<?php echo $block->escapeHtml($block->getValue()); ?>"
title="<?php echo $block->escapeHtml($block->getFieldLabel()); ?>"
class="input-text"
<?php /* @escapeNotVerified */ echo $block->escapeHtml($block->getFieldParams()); ?>
<?php if($block->isRequired()) echo 'data-validate="{required:true}"' ?>
/>
</div>
</div>
SandyAddressCustomAttributesSetupInstallData
<?php
namespace SandyAddressCustomAttributesSetup;
use MagentoFrameworkSetupInstallDataInterface;
use MagentoFrameworkSetupModuleDataSetupInterface;
use MagentoCustomerModelCustomer;
use MagentoFrameworkSetupModuleContextInterface;
use MagentoCustomerSetupCustomerSetupFactory;
class InstallData implements InstallDataInterface
{
private $customerSetupFactory;
/**
* Constructor
*
* @param MagentoCustomerSetupCustomerSetupFactory $customerSetupFactory
*/
public function __construct(
CustomerSetupFactory $customerSetupFactory
) {
$this->customerSetupFactory = $customerSetupFactory;
}
/**
* {@inheritdoc}
*/
public function install(
ModuleDataSetupInterface $setup,
ModuleContextInterface $context
) {
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerSetup->addAttribute(MagentoCustomerModelIndexerAddressAttributeProvider::ENTITY, 'comment', [
'label' => 'Comment',
'input' => 'textarea',
'type' => 'text',
'source' => '',
'required' => false,
'position' => 1001,
'visible' => true,
'system' => false,
'is_used_in_grid' => false,
'is_visible_in_grid' => false,
'is_filterable_in_grid' => false,
'is_searchable_in_grid' => false,
'backend' => ''
]);
$attribute = $customerSetup->getEavConfig()->getAttribute('customer_address', 'comment')
->addData(['used_in_forms' => [
'adminhtml_customer_address',
'customer_address_edit',
'customer_register_address'
]]);
$attribute->save();
}
}
magento2.3 customer-attribute customer-address
add a comment |
I have created a custom attribute for customer address. I can see the attribute on admin, I can insert value on frontend, the value saved successfully (and it displayed in address preview) however if I try to edit the address, no value appears.
This is my code. I will appreciate any help.
SandyAddressCustomAttributesBlockCustomerAddressFormEditComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerAddressFormEdit;
use MagentoCustomerApiAddressRepositoryInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkViewElementTemplate;
use MagentoCustomerApiDataAddressInterfaceFactory;
use MagentoCustomerModelSession;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressInterface */
private $address;
/** @var AddressRepositoryInterface */
private $addressRepository;
/** @var AddressInterfaceFactory */
private $addressFactory;
/** @var Session */
private $customerSession;
/**
* Comment Constructor
* @param Template|Context $context
* @param AddressRepositoryInterface $addressRepository
* @param AddressInterfaceFactory $addressFactory
* @param Session $session
* @param array $data
*/
public function __construct(
Context $context,
AddressRepositoryInterface $addressRepository,
AddressInterfaceFactory $addressFactory,
Session $session,
array $data =
)
{
parent::__construct($context, $data);
$this->addressRepository = $addressRepository;
$this->addressFactory = $addressFactory;
$this->customerSession = $session;
}
protected function _prepareLayout()
{
$addressId = $this->getRequest()->getParam('id');
if ($addressId) {
try {
$this->address = $this->addressRepository->getById($addressId);
if ($this->address->getCustomerId() != $this->customerSession->getCustomerId())
$this->address = null;
} catch (NoSuchEntityException $exception) {
$this->address = null;
}
}
if (null === $this->address)
$this->address = $this->addressFactory->create();
return parent::_prepareLayout();
}
/**
* @return string
* @throws MagentoFrameworkExceptionLocalizedException
*/
protected function _toHtml()
{
$customWidgetBlock = $this->getLayout()->createBlock(
'SandyAddressCustomAttributesBlockCustomerWidgetComment'
);
$customWidgetBlock->setAddress($this->address);
return $customWidgetBlock->toHtml();
}
}
SandyAddressCustomAttributesBlockCustomerWidgetComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerWidget;
use MagentoCustomerApiAddressMetadataInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkViewElementTemplate;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkPhrase;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressMetadataInterface */
private $addressMetadata;
protected function _construct()
{
parent::_construct();
$this->setTemplate('widget/comment.phtml');
}
/**
* Comment constructor.
* @param Context $context
* @param AddressMetadataInterface $addressMetadata
* @param array $data
*/
public function __construct(
Context $context,
AddressMetadataInterface $addressMetadata,
array $data =
)
{
parent::__construct($context, $data);
$this->addressMetadata = $addressMetadata;
}
/**
* @return bool
*/
public function isRequired()
{
return $this->getAttribute()
? $this->getAttribute()->isRequired()
: false;
}
/**
* @return string
*/
public function getFieldId()
{
return 'comment';
}
/**
* @return Phrase|string
*/
public function getFieldLabel()
{
return $this->getAttribute()
? $this->getAttribute()->getFrontendLabel()
: __('Comment');
}
/**
* @return string
*/
public function getFieldName()
{
return 'comment';
}
public function getSortOrder()
{
return $this->getAttribute()->getSortOrder();
}
/**
* @return string|null
*/
public function getValue()
{
/** @var AddressInterface $address */
$address = $this->getAddress();
if ($address instanceof AddressInterface) {
return $address->getCustomAttribute('comment')
? $address->getCustomAttribute('comment')->getValue()
: null;
}
return null;
}
private function getAttribute()
{
try {
$attribute = $this->addressMetadata->getAttributeMetadata('comment');
} catch (NoSuchEntityException $exception) {
$attribute = null;
}
return $attribute;
}
}
SandyAddressCustomAttributesPluginCustomerAddressEditPlugin.php
<?php
namespace SandyAddressCustomAttributesPluginCustomer;
use MagentoFrameworkViewLayoutInterface;
class AddressEditPlugin
{
/** @var LayoutInterface */
private $layout;
public function __construct(
LayoutInterface $layout
)
{
$this->layout = $layout;
}
/**
* @param MagentoCustomerBlockAddressEdit $edit
* @param $result
* @return string
*/
public function afterGetNameBlockHtml(
MagentoCustomerBlockAddressEdit $edit,
$result
)
{
$customBlock = $this->layout->createBlock(
'SandyAddressCustomAttributesBlockCustomerAddressFormEditComment',
'sandy_address_custom_attributes_comment'
);
return $result . $customBlock->toHtml();
}
}
view/frontend/templates/widget/comment.phtml
<?php /** @var SandyAddressCustomAttributesBlockCustomerWidgetComment $block */ ?>
<div class="field comment <?php if($block->isRequired()) echo ' required'; echo $block->escapeHtml($block->getSortOrder());?>">
<label class="label" for="<?php echo $block->escapeHtml($block->getFieldId()); ?>">
<span>
<?php echo $block->escapeHtml($block->getFieldLabel()); ?>
</span>
</label>
<div class="control">
<input type="text"
id="<?php echo $block->escapeHtml($block->getFieldId()); ?>"
name="<?php echo $block->escapeHtml($block->getFieldName()); ?>"
value="<?php echo $block->escapeHtml($block->getValue()); ?>"
title="<?php echo $block->escapeHtml($block->getFieldLabel()); ?>"
class="input-text"
<?php /* @escapeNotVerified */ echo $block->escapeHtml($block->getFieldParams()); ?>
<?php if($block->isRequired()) echo 'data-validate="{required:true}"' ?>
/>
</div>
</div>
SandyAddressCustomAttributesSetupInstallData
<?php
namespace SandyAddressCustomAttributesSetup;
use MagentoFrameworkSetupInstallDataInterface;
use MagentoFrameworkSetupModuleDataSetupInterface;
use MagentoCustomerModelCustomer;
use MagentoFrameworkSetupModuleContextInterface;
use MagentoCustomerSetupCustomerSetupFactory;
class InstallData implements InstallDataInterface
{
private $customerSetupFactory;
/**
* Constructor
*
* @param MagentoCustomerSetupCustomerSetupFactory $customerSetupFactory
*/
public function __construct(
CustomerSetupFactory $customerSetupFactory
) {
$this->customerSetupFactory = $customerSetupFactory;
}
/**
* {@inheritdoc}
*/
public function install(
ModuleDataSetupInterface $setup,
ModuleContextInterface $context
) {
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerSetup->addAttribute(MagentoCustomerModelIndexerAddressAttributeProvider::ENTITY, 'comment', [
'label' => 'Comment',
'input' => 'textarea',
'type' => 'text',
'source' => '',
'required' => false,
'position' => 1001,
'visible' => true,
'system' => false,
'is_used_in_grid' => false,
'is_visible_in_grid' => false,
'is_filterable_in_grid' => false,
'is_searchable_in_grid' => false,
'backend' => ''
]);
$attribute = $customerSetup->getEavConfig()->getAttribute('customer_address', 'comment')
->addData(['used_in_forms' => [
'adminhtml_customer_address',
'customer_address_edit',
'customer_register_address'
]]);
$attribute->save();
}
}
magento2.3 customer-attribute customer-address
I have created a custom attribute for customer address. I can see the attribute on admin, I can insert value on frontend, the value saved successfully (and it displayed in address preview) however if I try to edit the address, no value appears.
This is my code. I will appreciate any help.
SandyAddressCustomAttributesBlockCustomerAddressFormEditComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerAddressFormEdit;
use MagentoCustomerApiAddressRepositoryInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkViewElementTemplate;
use MagentoCustomerApiDataAddressInterfaceFactory;
use MagentoCustomerModelSession;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressInterface */
private $address;
/** @var AddressRepositoryInterface */
private $addressRepository;
/** @var AddressInterfaceFactory */
private $addressFactory;
/** @var Session */
private $customerSession;
/**
* Comment Constructor
* @param Template|Context $context
* @param AddressRepositoryInterface $addressRepository
* @param AddressInterfaceFactory $addressFactory
* @param Session $session
* @param array $data
*/
public function __construct(
Context $context,
AddressRepositoryInterface $addressRepository,
AddressInterfaceFactory $addressFactory,
Session $session,
array $data =
)
{
parent::__construct($context, $data);
$this->addressRepository = $addressRepository;
$this->addressFactory = $addressFactory;
$this->customerSession = $session;
}
protected function _prepareLayout()
{
$addressId = $this->getRequest()->getParam('id');
if ($addressId) {
try {
$this->address = $this->addressRepository->getById($addressId);
if ($this->address->getCustomerId() != $this->customerSession->getCustomerId())
$this->address = null;
} catch (NoSuchEntityException $exception) {
$this->address = null;
}
}
if (null === $this->address)
$this->address = $this->addressFactory->create();
return parent::_prepareLayout();
}
/**
* @return string
* @throws MagentoFrameworkExceptionLocalizedException
*/
protected function _toHtml()
{
$customWidgetBlock = $this->getLayout()->createBlock(
'SandyAddressCustomAttributesBlockCustomerWidgetComment'
);
$customWidgetBlock->setAddress($this->address);
return $customWidgetBlock->toHtml();
}
}
SandyAddressCustomAttributesBlockCustomerWidgetComment.php
<?php
namespace SandyAddressCustomAttributesBlockCustomerWidget;
use MagentoCustomerApiAddressMetadataInterface;
use MagentoCustomerApiDataAddressInterface;
use MagentoFrameworkViewElementTemplate;
use MagentoFrameworkExceptionNoSuchEntityException;
use MagentoFrameworkPhrase;
use MagentoFrameworkViewElementTemplateContext;
class Comment extends Template
{
/** @var AddressMetadataInterface */
private $addressMetadata;
protected function _construct()
{
parent::_construct();
$this->setTemplate('widget/comment.phtml');
}
/**
* Comment constructor.
* @param Context $context
* @param AddressMetadataInterface $addressMetadata
* @param array $data
*/
public function __construct(
Context $context,
AddressMetadataInterface $addressMetadata,
array $data =
)
{
parent::__construct($context, $data);
$this->addressMetadata = $addressMetadata;
}
/**
* @return bool
*/
public function isRequired()
{
return $this->getAttribute()
? $this->getAttribute()->isRequired()
: false;
}
/**
* @return string
*/
public function getFieldId()
{
return 'comment';
}
/**
* @return Phrase|string
*/
public function getFieldLabel()
{
return $this->getAttribute()
? $this->getAttribute()->getFrontendLabel()
: __('Comment');
}
/**
* @return string
*/
public function getFieldName()
{
return 'comment';
}
public function getSortOrder()
{
return $this->getAttribute()->getSortOrder();
}
/**
* @return string|null
*/
public function getValue()
{
/** @var AddressInterface $address */
$address = $this->getAddress();
if ($address instanceof AddressInterface) {
return $address->getCustomAttribute('comment')
? $address->getCustomAttribute('comment')->getValue()
: null;
}
return null;
}
private function getAttribute()
{
try {
$attribute = $this->addressMetadata->getAttributeMetadata('comment');
} catch (NoSuchEntityException $exception) {
$attribute = null;
}
return $attribute;
}
}
SandyAddressCustomAttributesPluginCustomerAddressEditPlugin.php
<?php
namespace SandyAddressCustomAttributesPluginCustomer;
use MagentoFrameworkViewLayoutInterface;
class AddressEditPlugin
{
/** @var LayoutInterface */
private $layout;
public function __construct(
LayoutInterface $layout
)
{
$this->layout = $layout;
}
/**
* @param MagentoCustomerBlockAddressEdit $edit
* @param $result
* @return string
*/
public function afterGetNameBlockHtml(
MagentoCustomerBlockAddressEdit $edit,
$result
)
{
$customBlock = $this->layout->createBlock(
'SandyAddressCustomAttributesBlockCustomerAddressFormEditComment',
'sandy_address_custom_attributes_comment'
);
return $result . $customBlock->toHtml();
}
}
view/frontend/templates/widget/comment.phtml
<?php /** @var SandyAddressCustomAttributesBlockCustomerWidgetComment $block */ ?>
<div class="field comment <?php if($block->isRequired()) echo ' required'; echo $block->escapeHtml($block->getSortOrder());?>">
<label class="label" for="<?php echo $block->escapeHtml($block->getFieldId()); ?>">
<span>
<?php echo $block->escapeHtml($block->getFieldLabel()); ?>
</span>
</label>
<div class="control">
<input type="text"
id="<?php echo $block->escapeHtml($block->getFieldId()); ?>"
name="<?php echo $block->escapeHtml($block->getFieldName()); ?>"
value="<?php echo $block->escapeHtml($block->getValue()); ?>"
title="<?php echo $block->escapeHtml($block->getFieldLabel()); ?>"
class="input-text"
<?php /* @escapeNotVerified */ echo $block->escapeHtml($block->getFieldParams()); ?>
<?php if($block->isRequired()) echo 'data-validate="{required:true}"' ?>
/>
</div>
</div>
SandyAddressCustomAttributesSetupInstallData
<?php
namespace SandyAddressCustomAttributesSetup;
use MagentoFrameworkSetupInstallDataInterface;
use MagentoFrameworkSetupModuleDataSetupInterface;
use MagentoCustomerModelCustomer;
use MagentoFrameworkSetupModuleContextInterface;
use MagentoCustomerSetupCustomerSetupFactory;
class InstallData implements InstallDataInterface
{
private $customerSetupFactory;
/**
* Constructor
*
* @param MagentoCustomerSetupCustomerSetupFactory $customerSetupFactory
*/
public function __construct(
CustomerSetupFactory $customerSetupFactory
) {
$this->customerSetupFactory = $customerSetupFactory;
}
/**
* {@inheritdoc}
*/
public function install(
ModuleDataSetupInterface $setup,
ModuleContextInterface $context
) {
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerSetup->addAttribute(MagentoCustomerModelIndexerAddressAttributeProvider::ENTITY, 'comment', [
'label' => 'Comment',
'input' => 'textarea',
'type' => 'text',
'source' => '',
'required' => false,
'position' => 1001,
'visible' => true,
'system' => false,
'is_used_in_grid' => false,
'is_visible_in_grid' => false,
'is_filterable_in_grid' => false,
'is_searchable_in_grid' => false,
'backend' => ''
]);
$attribute = $customerSetup->getEavConfig()->getAttribute('customer_address', 'comment')
->addData(['used_in_forms' => [
'adminhtml_customer_address',
'customer_address_edit',
'customer_register_address'
]]);
$attribute->save();
}
}
magento2.3 customer-attribute customer-address
magento2.3 customer-attribute customer-address
edited 1 min ago


HIren Kadivar
716214
716214
asked 10 hours ago


Sandy LampropoulouSandy Lampropoulou
1
1
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "479"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmagento.stackexchange.com%2fquestions%2f264766%2fcustom-address-attribute-issue-on-display-value-magento-2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Magento Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmagento.stackexchange.com%2fquestions%2f264766%2fcustom-address-attribute-issue-on-display-value-magento-2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
L6v7,vhzT9GOG3vBopZXLWDT,f4t TxztPqLOEiKB72,mTWETtud2LjN DA5c8oMKzo0Y2tV K9o,mJ03c28TWkK281F2 QC1H O