Magento227 Maketplace Coding Standard Issue
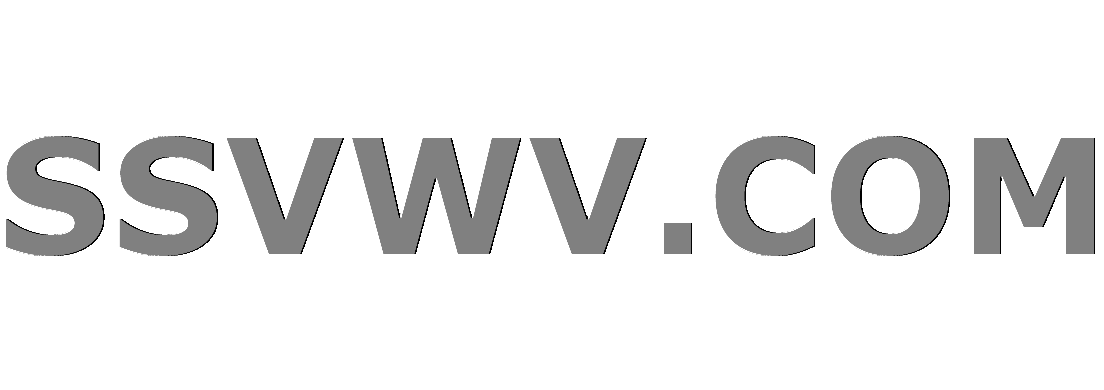
Multi tool use
When I run: phpcs /path/to/module --standard=MEQP2
It gives me the following error
PHP Fatal error: Trait 'MEQPUtilsHelper' not found in
/opt/marketplace-eqp/MEQP2/Sniffs/Classes/NameResolutionSniff.php on
line 23
phpcs -i
The installed coding standards are PSR1, PEAR, Zend, MySource, PSR12, PSR2, Squiz, MEQP2 and MEQP1
class NameResolutionSniff
<?php
/**
* Copyright © Magento. All rights reserved.
* See COPYING.txt for license details.
*/
namespace MEQP2SniffsClasses;
use PHP_CodeSnifferConfig;
use PHP_CodeSnifferFilesFile;
use PHP_CodeSnifferSniffsSniff;
use MEQPUtilsHelper;
/**
* Class NameResolutionSniff
* Dynamic sniff that detects the use of literal class and interface names.
* Requires 'm2-path' to be configured.
*/
class NameResolutionSniff implements Sniff
{
/**
* Include Helper trait.
*/
use Helper;
/**
* Violation severity.
*
* @var int
*/
protected $severity = 8;
/**
* String representation of warning.
*
* @var string
*/
protected $warningMessage = 'Literal namespace detected. Use ::class notation instead.';
/**
* Warning violation code.
*
* @var string
*/
protected $warningCode = 'LiteralNamespaceFound';
/**
* Literal namespace pattern.
*
* @var string
*/
private $literalNamespacePattern = '/^[\]{0,2}[A-Z][A-Za-z0-9]+([\]{1,2}[A-Z][A-Za-z0-9]+){2,}(?!\+)$/';
/**
* Class names from current file.
*
* @var array
*/
private $classNames = ;
/**
* A bootstrap of Magento application.
*
* @var MagentoFrameworkAppBootstrap
*/
private $bootstrap;
/**
* @inheritdoc
*/
public function register()
{
return [
T_CONSTANT_ENCAPSED_STRING,
T_DOUBLE_QUOTED_STRING,
];
}
/**
* @inheritdoc
*/
public function process(File $sourceFile, $stackPtr)
{
if (Config::getConfigData('m2-path') === null ||
$sourceFile->findPrevious([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr - 1, null, false, null, true) ||
$sourceFile->findNext([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr + 1, null, false, null, true)
) {
return;
}
$tokens = $sourceFile->getTokens();
$content = trim($tokens[$stackPtr]['content'], ""'");
if (preg_match($this->literalNamespacePattern, $content) === 1) {
if (!$this->bootstrap) {
$this->bootstrap = $this->getBootstrap();
}
if ($this->classExists($content)) {
$sourceFile->addWarning($this->warningMessage, $stackPtr, $this->warningCode, , $this->severity);
}
}
}
/**
* Checks if class exists by class name.
*
* @param string $className
* @return bool
*/
private function classExists($className)
{
if (!array_key_exists($className, $this->classNames)) {
$this->classNames[$className] = class_exists($className) || interface_exists($className);
}
return $this->classNames[$className];
}
}
and line no 23 is :
use Helper;
Any idea how to fix this issue?
magento2.2 marketplace coding-standards
add a comment |
When I run: phpcs /path/to/module --standard=MEQP2
It gives me the following error
PHP Fatal error: Trait 'MEQPUtilsHelper' not found in
/opt/marketplace-eqp/MEQP2/Sniffs/Classes/NameResolutionSniff.php on
line 23
phpcs -i
The installed coding standards are PSR1, PEAR, Zend, MySource, PSR12, PSR2, Squiz, MEQP2 and MEQP1
class NameResolutionSniff
<?php
/**
* Copyright © Magento. All rights reserved.
* See COPYING.txt for license details.
*/
namespace MEQP2SniffsClasses;
use PHP_CodeSnifferConfig;
use PHP_CodeSnifferFilesFile;
use PHP_CodeSnifferSniffsSniff;
use MEQPUtilsHelper;
/**
* Class NameResolutionSniff
* Dynamic sniff that detects the use of literal class and interface names.
* Requires 'm2-path' to be configured.
*/
class NameResolutionSniff implements Sniff
{
/**
* Include Helper trait.
*/
use Helper;
/**
* Violation severity.
*
* @var int
*/
protected $severity = 8;
/**
* String representation of warning.
*
* @var string
*/
protected $warningMessage = 'Literal namespace detected. Use ::class notation instead.';
/**
* Warning violation code.
*
* @var string
*/
protected $warningCode = 'LiteralNamespaceFound';
/**
* Literal namespace pattern.
*
* @var string
*/
private $literalNamespacePattern = '/^[\]{0,2}[A-Z][A-Za-z0-9]+([\]{1,2}[A-Z][A-Za-z0-9]+){2,}(?!\+)$/';
/**
* Class names from current file.
*
* @var array
*/
private $classNames = ;
/**
* A bootstrap of Magento application.
*
* @var MagentoFrameworkAppBootstrap
*/
private $bootstrap;
/**
* @inheritdoc
*/
public function register()
{
return [
T_CONSTANT_ENCAPSED_STRING,
T_DOUBLE_QUOTED_STRING,
];
}
/**
* @inheritdoc
*/
public function process(File $sourceFile, $stackPtr)
{
if (Config::getConfigData('m2-path') === null ||
$sourceFile->findPrevious([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr - 1, null, false, null, true) ||
$sourceFile->findNext([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr + 1, null, false, null, true)
) {
return;
}
$tokens = $sourceFile->getTokens();
$content = trim($tokens[$stackPtr]['content'], ""'");
if (preg_match($this->literalNamespacePattern, $content) === 1) {
if (!$this->bootstrap) {
$this->bootstrap = $this->getBootstrap();
}
if ($this->classExists($content)) {
$sourceFile->addWarning($this->warningMessage, $stackPtr, $this->warningCode, , $this->severity);
}
}
}
/**
* Checks if class exists by class name.
*
* @param string $className
* @return bool
*/
private function classExists($className)
{
if (!array_key_exists($className, $this->classNames)) {
$this->classNames[$className] = class_exists($className) || interface_exists($className);
}
return $this->classNames[$className];
}
}
and line no 23 is :
use Helper;
Any idea how to fix this issue?
magento2.2 marketplace coding-standards
add a comment |
When I run: phpcs /path/to/module --standard=MEQP2
It gives me the following error
PHP Fatal error: Trait 'MEQPUtilsHelper' not found in
/opt/marketplace-eqp/MEQP2/Sniffs/Classes/NameResolutionSniff.php on
line 23
phpcs -i
The installed coding standards are PSR1, PEAR, Zend, MySource, PSR12, PSR2, Squiz, MEQP2 and MEQP1
class NameResolutionSniff
<?php
/**
* Copyright © Magento. All rights reserved.
* See COPYING.txt for license details.
*/
namespace MEQP2SniffsClasses;
use PHP_CodeSnifferConfig;
use PHP_CodeSnifferFilesFile;
use PHP_CodeSnifferSniffsSniff;
use MEQPUtilsHelper;
/**
* Class NameResolutionSniff
* Dynamic sniff that detects the use of literal class and interface names.
* Requires 'm2-path' to be configured.
*/
class NameResolutionSniff implements Sniff
{
/**
* Include Helper trait.
*/
use Helper;
/**
* Violation severity.
*
* @var int
*/
protected $severity = 8;
/**
* String representation of warning.
*
* @var string
*/
protected $warningMessage = 'Literal namespace detected. Use ::class notation instead.';
/**
* Warning violation code.
*
* @var string
*/
protected $warningCode = 'LiteralNamespaceFound';
/**
* Literal namespace pattern.
*
* @var string
*/
private $literalNamespacePattern = '/^[\]{0,2}[A-Z][A-Za-z0-9]+([\]{1,2}[A-Z][A-Za-z0-9]+){2,}(?!\+)$/';
/**
* Class names from current file.
*
* @var array
*/
private $classNames = ;
/**
* A bootstrap of Magento application.
*
* @var MagentoFrameworkAppBootstrap
*/
private $bootstrap;
/**
* @inheritdoc
*/
public function register()
{
return [
T_CONSTANT_ENCAPSED_STRING,
T_DOUBLE_QUOTED_STRING,
];
}
/**
* @inheritdoc
*/
public function process(File $sourceFile, $stackPtr)
{
if (Config::getConfigData('m2-path') === null ||
$sourceFile->findPrevious([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr - 1, null, false, null, true) ||
$sourceFile->findNext([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr + 1, null, false, null, true)
) {
return;
}
$tokens = $sourceFile->getTokens();
$content = trim($tokens[$stackPtr]['content'], ""'");
if (preg_match($this->literalNamespacePattern, $content) === 1) {
if (!$this->bootstrap) {
$this->bootstrap = $this->getBootstrap();
}
if ($this->classExists($content)) {
$sourceFile->addWarning($this->warningMessage, $stackPtr, $this->warningCode, , $this->severity);
}
}
}
/**
* Checks if class exists by class name.
*
* @param string $className
* @return bool
*/
private function classExists($className)
{
if (!array_key_exists($className, $this->classNames)) {
$this->classNames[$className] = class_exists($className) || interface_exists($className);
}
return $this->classNames[$className];
}
}
and line no 23 is :
use Helper;
Any idea how to fix this issue?
magento2.2 marketplace coding-standards
When I run: phpcs /path/to/module --standard=MEQP2
It gives me the following error
PHP Fatal error: Trait 'MEQPUtilsHelper' not found in
/opt/marketplace-eqp/MEQP2/Sniffs/Classes/NameResolutionSniff.php on
line 23
phpcs -i
The installed coding standards are PSR1, PEAR, Zend, MySource, PSR12, PSR2, Squiz, MEQP2 and MEQP1
class NameResolutionSniff
<?php
/**
* Copyright © Magento. All rights reserved.
* See COPYING.txt for license details.
*/
namespace MEQP2SniffsClasses;
use PHP_CodeSnifferConfig;
use PHP_CodeSnifferFilesFile;
use PHP_CodeSnifferSniffsSniff;
use MEQPUtilsHelper;
/**
* Class NameResolutionSniff
* Dynamic sniff that detects the use of literal class and interface names.
* Requires 'm2-path' to be configured.
*/
class NameResolutionSniff implements Sniff
{
/**
* Include Helper trait.
*/
use Helper;
/**
* Violation severity.
*
* @var int
*/
protected $severity = 8;
/**
* String representation of warning.
*
* @var string
*/
protected $warningMessage = 'Literal namespace detected. Use ::class notation instead.';
/**
* Warning violation code.
*
* @var string
*/
protected $warningCode = 'LiteralNamespaceFound';
/**
* Literal namespace pattern.
*
* @var string
*/
private $literalNamespacePattern = '/^[\]{0,2}[A-Z][A-Za-z0-9]+([\]{1,2}[A-Z][A-Za-z0-9]+){2,}(?!\+)$/';
/**
* Class names from current file.
*
* @var array
*/
private $classNames = ;
/**
* A bootstrap of Magento application.
*
* @var MagentoFrameworkAppBootstrap
*/
private $bootstrap;
/**
* @inheritdoc
*/
public function register()
{
return [
T_CONSTANT_ENCAPSED_STRING,
T_DOUBLE_QUOTED_STRING,
];
}
/**
* @inheritdoc
*/
public function process(File $sourceFile, $stackPtr)
{
if (Config::getConfigData('m2-path') === null ||
$sourceFile->findPrevious([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr - 1, null, false, null, true) ||
$sourceFile->findNext([T_STRING_CONCAT, T_CONCAT_EQUAL], $stackPtr + 1, null, false, null, true)
) {
return;
}
$tokens = $sourceFile->getTokens();
$content = trim($tokens[$stackPtr]['content'], ""'");
if (preg_match($this->literalNamespacePattern, $content) === 1) {
if (!$this->bootstrap) {
$this->bootstrap = $this->getBootstrap();
}
if ($this->classExists($content)) {
$sourceFile->addWarning($this->warningMessage, $stackPtr, $this->warningCode, , $this->severity);
}
}
}
/**
* Checks if class exists by class name.
*
* @param string $className
* @return bool
*/
private function classExists($className)
{
if (!array_key_exists($className, $this->classNames)) {
$this->classNames[$className] = class_exists($className) || interface_exists($className);
}
return $this->classNames[$className];
}
}
and line no 23 is :
use Helper;
Any idea how to fix this issue?
magento2.2 marketplace coding-standards
magento2.2 marketplace coding-standards
edited 2 mins ago
Verdu
asked 7 mins ago


VerduVerdu
1,049522
1,049522
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "479"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmagento.stackexchange.com%2fquestions%2f257771%2fmagento227-maketplace-coding-standard-issue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Magento Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmagento.stackexchange.com%2fquestions%2f257771%2fmagento227-maketplace-coding-standard-issue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mnRfcmyQhVgc1oFiUpY 7kOB qzz,PFblDx